Class exiftool.ExifTool
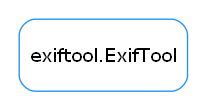
Run the exiftool command-line tool and communicate with it.
Use common_args
to enable/disable print conversion by specifying/omitting -n
, respectively.
This determines whether exiftool should perform print conversion,
which prints values in a human-readable way but
may be slower. If print conversion is enabled, appending #
to a tag
name disables the print conversion for this particular tag.
See Exiftool print conversion FAQ for more details.
Some methods of this class are only available after calling
run()
, which will actually launch the exiftool subprocess.
To avoid leaving the subprocess running, make sure to call
terminate()
method when finished using the instance.
This method will also be implicitly called when the instance is
garbage collected, but there are circumstance when this won’t ever
happen, so you should not rely on the implicit process
termination. Subprocesses won’t be automatically terminated if
the parent process exits, so a leaked subprocess will stay around
until manually killed.
A convenient way to make sure that the subprocess is terminated is
to use the ExifTool
instance as a context manager:
with ExifTool() as et:
...
Warning
Note that options and parameters are not checked. There is no error handling or validation of options passed to exiftool.
Nonsensical options are mostly silently ignored by exiftool, so there’s not much that can be done in that regard. You should avoid passing non-existent files to any of the methods, since this will lead to undefined behaviour.
- param executable:
Specify file name of the exiftool executable if it is in your
PATH
. Otherwise, specify the full path to theexiftool
executable.Passed directly into
executable
property.Note
The default value
exiftool.constants.DEFAULT_EXECUTABLE
will only work if the executable is in yourPATH
.- type executable:
str, or None to use default
- param common_args:
Pass in additional parameters for the stay-open instance of exiftool.
Defaults to
["-G", "-n"]
as this is the most common use case.-G
(groupName level 1 enabled) separates the output with groupName:tag to disambiguate same-named tags under different groups.-n
(print conversion disabled) improves the speed and consistency of output, and is more machine-parsable
Passed directly into
common_args
property.Note
Depending on your use case, there may be other useful grouping levels and options. Search Phil Harvey’s exiftool documentation for groupNames and groupHeadings to get more info.
- type common_args:
list of str, or None.
- param bool win_shell:
(Windows only) Minimizes the exiftool process.
Note
This parameter may be deprecated in the future
- param config_file:
File path to
-config
parameter when starting exiftool process.Passed directly into
config_file
property.- type config_file:
str, Path, or None
- param encoding:
Specify encoding to be used when communicating with exiftool process. By default, will use
locale.getpreferredencoding()
Passed directly into
encoding
property.- param logger:
Set a custom logger to log status and debug messages to.
Passed directly into
logger
property.
- exiftool.ExifTool.__init__(executable=None, common_args=['-G', '-n'], win_shell=False, config_file=None, encoding=None, logger=None)
- Parameters:
executable (str, or None to use default) –
Specify file name of the exiftool executable if it is in your
PATH
. Otherwise, specify the full path to theexiftool
executable.Passed directly into
executable
property.Note
The default value
exiftool.constants.DEFAULT_EXECUTABLE
will only work if the executable is in yourPATH
.common_args (list of str, or None.) –
Pass in additional parameters for the stay-open instance of exiftool.
Defaults to
["-G", "-n"]
as this is the most common use case.-G
(groupName level 1 enabled) separates the output with groupName:tag to disambiguate same-named tags under different groups.-n
(print conversion disabled) improves the speed and consistency of output, and is more machine-parsable
Passed directly into
common_args
property.Note
Depending on your use case, there may be other useful grouping levels and options. Search Phil Harvey’s exiftool documentation for groupNames and groupHeadings to get more info.
win_shell (bool) –
(Windows only) Minimizes the exiftool process.
Note
This parameter may be deprecated in the future
config_file (str, Path, or None) –
File path to
-config
parameter when starting exiftool process.Passed directly into
config_file
property.encoding (Optional[str]) –
Specify encoding to be used when communicating with exiftool process. By default, will use
locale.getpreferredencoding()
Passed directly into
encoding
property.logger –
Set a custom logger to log status and debug messages to.
Passed directly into
logger
property.
- Return type:
None
- property exiftool.ExifTool.block_size
Block size for communicating with exiftool subprocess. Used when reading from the I/O pipe.
- Getter:
Returns current block size
- Setter:
Set a new block_size. Does basic error checking to make sure > 0.
- Raises:
ValueError – If new block size is invalid
- Type:
int
- Return type:
int
- property exiftool.ExifTool.common_args
Common Arguments executed with every command passed to exiftool subprocess
This is the parameter -common_args that is passed when the exiftool process is STARTED
Read Phil Harvey’s ExifTool documentation to get further information on what options are available / how to use them.
- Getter:
Returns current common_args list
- Setter:
If
None
is passed in, sets common_args to[]
. Otherwise, sets the given list without any validation.Warning
No validation is done on the arguments list. It is passed verbatim to exiftool. Invalid options or combinations may result in undefined behavior.
Note
Setting is only available when exiftool process is not running.
- Raises:
ExifToolRunning – If attempting to set while running (
running
== True)TypeError – If setting is not a list
- Type:
list[str], None
- Return type:
Optional[List[str]]
- property exiftool.ExifTool.config_file
Path to config file.
See ExifTool documentation for -config for more details.
- Getter:
Returns current config file path, or None if not set
- Setter:
File existence is checked when setting parameter
Set to
None
to disable the-config
parameter when starting exiftoolSet to
""
has special meaning and disables loading of the default config file. See ExifTool documentation for -config for more info.
Note
Currently file is checked for existence when setting. It is not checked when starting process.
- Raises:
ExifToolRunning – If attempting to set while running (
running
== True)- Type:
str, Path, None
- Return type:
Optional[Union[str, pathlib.Path]]
- property exiftool.ExifTool.encoding
Encoding of Popen() communication with exiftool process.
- Getter:
Returns current encoding setting
- Setter:
Set a new encoding.
If new_encoding is None, will detect it from
locale.getpreferredencoding(do_setlocale=False)
(do_setlocale is set to False as not to affect the caller).Default to
utf-8
if nothing is returned bygetpreferredencoding
Warning
Property setter does NOT validate the encoding for validity. It is passed verbatim into subprocess.Popen()
Note
Setting is only available when exiftool process is not running.
- Raises:
ExifToolRunning – If attempting to set while running (
running
== True)- Return type:
Optional[str]
- property exiftool.ExifTool.executable
Path to exiftool executable.
- Getter:
Returns current exiftool path
- Setter:
Specify just the executable name, or an absolute path to the executable. If path given is not absolute, searches environment
PATH
.Note
Setting is only available when exiftool process is not running.
- Raises:
ExifToolRunning – If attempting to set while running (
running
== True)- Type:
str, Path
- Return type:
Union[str, pathlib.Path]
- exiftool.ExifTool.execute(*params, raw_bytes=False)
Execute the given batch of parameters with exiftool.
This method accepts any number of parameters and sends them to the attached
exiftool
subprocess. The process must be running, otherwiseexiftool.exceptions.ExifToolNotRunning
is raised. The final-execute
necessary to actually run the batch is appended automatically; see the documentation ofrun()
for the common options. Theexiftool
output is read up to the end-of-output sentinel and returned as astr
decoded based on the currently setencoding
, excluding the sentinel.The parameters must be of type
str
orbytes
.str
parameters are encoded to bytes automatically using theencoding
property. For filenames, this should be the system’s filesystem encoding.bytes
parameters are untouched and passed directly toexiftool
.Note
This is the core method to interface with the
exiftool
subprocess.No processing is done on the input or output.
- Parameters:
params (one or more string/bytes parameters) –
One or more parameters to send to the
exiftool
subprocess.Typically passed in via Unpacking Argument Lists
Note
The parameters to this function must be type
str
orbytes
.raw_bytes (bool) – If True, returns bytes. Default behavior returns a str
- Returns:
STDOUT is returned by the method call, and is also set in
last_stdout
STDERR is set in
last_stderr
Exit Status of the command is set in
last_status
- Raises:
ExifToolNotRunning – If attempting to execute when not running (
running
== False)ExifToolVersionError – If unexpected text was returned from the command while parsing out the sentinels
UnicodeDecodeError – If the
encoding
is not specified properly, it may be possible for.decode()
method to raise this errorTypeError – If
params
argument is notstr
orbytes
- Return type:
Union[str, bytes]
- exiftool.ExifTool.execute_json(*params)
Execute the given batch of parameters and parse the JSON output.
This method is similar to
execute()
. It automatically adds the parameter-j
to request JSON output fromexiftool
and parses the output.The return value is a list of dictionaries, mapping tag names to the corresponding values. All keys are strings. The values can have multiple types. Each dictionary contains the name of the file it corresponds to in the key
"SourceFile"
.Note
By default, the tag names include the group name in the format <group>:<tag> (if using the
-G
option).You can adjust the output structure with various exiftool options.
Warning
This method does not verify the exit status code returned by exiftool. That is left up to the caller.
This will mimic exiftool’s default method of operation “continue on error” and “best attempt” to complete commands given.
If you want automated error checking, use
exiftool.ExifToolHelper
- Parameters:
params (one or more string/bytes parameters) –
One or more parameters to send to the
exiftool
subprocess.Typically passed in via Unpacking Argument Lists
Note
The parameters to this function must be type
str
orbytes
.- Returns:
Valid JSON parsed into a Python list of dicts
- Raises:
If exiftool did not return any STDOUT
Note
This is not necessarily an exiftool error, but rather a programmer error.
For example, setting tags can cause this behavior.
If you are executing a command and expect no output, use
execute()
instead.If exiftool returned STDOUT which is invalid JSON.
Note
This is not necessarily an exiftool error, but rather a programmer error.
For example,
-w
can cause this behavior.If you are executing a command and expect non-JSON output, use
execute()
instead.
- Return type:
List
- property exiftool.ExifTool.last_status
Exit Status Code
for most recent result from execute()Note
This property can be read at any time, and is not dependent on running state of ExifTool.
It is INTENTIONALLY NOT CLEARED on exiftool termination, to allow for executing a command and terminating, but still having the result available.
- Return type:
Optional[int]
- property exiftool.ExifTool.last_stderr
STDERR
for most recent result from execute()Note
The return type can be either
str
orbytes
.If the most recent call to execute()
raw_bytes=True
, then this will returnbytes
. Otherwise this will bestr
.Note
This property can be read at any time, and is not dependent on running state of ExifTool.
It is INTENTIONALLY NOT CLEARED on exiftool termination, to allow for executing a command and terminating, but still having the result available.
- Return type:
Optional[Union[str, bytes]]
- property exiftool.ExifTool.last_stdout
STDOUT
for most recent result from execute()Note
The return type can be either str or bytes.
If the most recent call to execute()
raw_bytes=True
, then this will returnbytes
. Otherwise this will bestr
.Note
This property can be read at any time, and is not dependent on running state of ExifTool.
It is INTENTIONALLY NOT CLEARED on exiftool termination, to allow for executing a command and terminating, but still having the result available.
- Return type:
Optional[Union[str, bytes]]
- exiftool.ExifTool.logger
Write-only property to set the class of logging.Logger
If this is set, then status messages will log out to the given class.
Note
This can be set and unset (set to
None
) at any time, regardless of whether the subprocess is running (running
== True) or not.- Setter:
Specify an object to log to. The class is not checked, but validation is done to ensure the object has callable methods
info
,warning
,error
,critical
,exception
.- Raises:
AttributeError – If object does not contain one or more of the required methods.
TypeError – If object contains those attributes, but one or more are non-callable methods.
- Type:
Object
- exiftool.ExifTool.run()
Start an exiftool subprocess in batch mode.
This method will issue a
UserWarning
if the subprocess is already running (running
== True). The process is started withcommon_args
as common arguments, which are automatically included in every command you run withexecute()
.You can override these default arguments with the
common_args
parameter in the constructor or settingcommon_args
before caalingrun()
.Note
If you have another executable named exiftool which isn’t Phil Harvey’s ExifTool, then you’re shooting yourself in the foot as there’s no error checking for that
- Raises:
FileNotFoundError – If exiftool is no longer found. Re-raised from subprocess.Popen()
OSError – Re-raised from subprocess.Popen()
ValueError – Re-raised from subprocess.Popen()
subproccess.CalledProcessError – Re-raised from subprocess.Popen()
RuntimeError – Popen() launched process but it died right away
ExifToolVersionError –
exiftool.constants.EXIFTOOL_MINIMUM_VERSION
not met. ExifTool process will be automatically terminated.
- Return type:
None
- property exiftool.ExifTool.running
Read-only property which indicates whether the exiftool subprocess is running or not.
- Getter:
Returns current running state
Note
This checks to make sure the process is still alive.
If the process has died since last running detection, this property will detect the state change and reset the status accordingly.
- Return type:
bool
- exiftool.ExifTool.set_json_loads(json_loads, **kwargs)
Advanced: Override default built-in
json.loads()
method. The method is only used once inexecute_json()
This allows using a different json string parser.
(Alternate json libraries typically provide faster speed than the built-in implementation, more supported features, and/or different behavior.)
Examples of json libraries: orjson, rapidjson, ujson, …
Note
This method is designed to be called the same way you would expect to call the provided
json_loads
method.Include any
kwargs
you would in the call.For example, to pass additional arguments to
json.loads()
:set_json_loads(json.loads, parse_float=str)
Note
This can be set at any time, regardless of whether the subprocess is running (
running
== True) or not.Warning
This setter does not check whether the method provided actually parses json. Undefined behavior or crashes may occur if used incorrectly
This is advanced configuration for specific use cases only.
For an example use case, see the FAQ
- Parameters:
json_loads (callable) – A callable method to replace built-in
json.loads
used inexecute_json()
kwargs – Parameters passed to the
json_loads
method call
- Raises:
TypeError – If
json_loads
is not callable- Return type:
None
- exiftool.ExifTool.terminate(timeout=30, _del=False)
Terminate the exiftool subprocess.
If the subprocess isn’t running, this method will throw a warning, and do nothing.
Note
There is a bug in CPython 3.8+ on Windows where terminate() does not work during
__del__()
See CPython issue starting a thread in __del__ hangs at interpreter shutdown for more info.
- Parameters:
timeout (int) –
_del (bool) –
- Return type:
None
- property exiftool.ExifTool.version
Read-only property which is the string returned by
exiftool -ver
The -ver command is ran once at process startup and cached.
- Getter:
Returns cached output of
exiftool -ver
- Raises:
ExifToolNotRunning – If attempting to read while not running (
running
== False)- Return type:
str