Class exiftool.ExifToolHelper
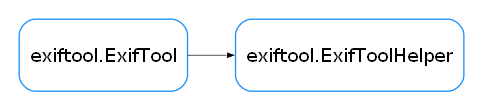
This class extends the low-level exiftool.ExifTool
class with ‘wrapper’/’helper’ functionality
It keeps low-level core functionality with the base class but extends helper functions in a separate class
- param bool auto_start:
Will automatically start the exiftool process on first command run, defaults to True
- param bool check_execute:
Will check the exit status (return code) of all commands. This catches some invalid commands passed to exiftool subprocess, defaults to True. See
check_execute
for more info.- param bool check_tag_names:
Will check the tag names provided to methods which work directly with tag names. This catches unintended uses and bugs, default to True. See
check_tag_names
for more info.- param kwargs:
All other parameters are passed directly to the super-class constructor:
exiftool.ExifTool.__init__()
- exiftool.ExifToolHelper.__init__(auto_start=True, check_execute=True, check_tag_names=True, **kwargs)
- Parameters:
auto_start (bool) – Will automatically start the exiftool process on first command run, defaults to True
check_execute (bool) – Will check the exit status (return code) of all commands. This catches some invalid commands passed to exiftool subprocess, defaults to True. See
check_execute
for more info.check_tag_names (bool) – Will check the tag names provided to methods which work directly with tag names. This catches unintended uses and bugs, default to True. See
check_tag_names
for more info.kwargs – All other parameters are passed directly to the super-class constructor:
exiftool.ExifTool.__init__()
- Return type:
None
- property exiftool.ExifToolHelper.auto_start
Read-only property. Gets the current setting passed into the constructor as to whether auto_start is enabled or not.
(There’s really no point to having this a read-write property, but allowing a read can be helpful at runtime to detect expected behavior.)
- Return type:
bool
- property exiftool.ExifToolHelper.check_execute
Flag to enable/disable checking exit status (return code) on execute
If enabled, will raise
exiftool.exceptions.ExifToolExecuteError
if a non-zero exit status is returned duringexecute()
Warning
While this property is provided to give callers an option to enable/disable error checking, it is generally NOT recommended to disable
check_execute
.If disabled, exiftool will fail silently, and hard-to-catch bugs may arise.
That said, there may be some use cases where continue-on-error behavior is desired. (Example: dump all exif in a directory with files which don’t all have the same tags, exiftool returns exit code 1 for unknown files, but results are valid for other files with those tags)
- Getter:
Returns current setting
- Setter:
Enable or Disable the check
Note
This settings can be changed any time and will only affect subsequent calls
- Type:
bool
- Return type:
bool
- property exiftool.ExifToolHelper.check_tag_names
Flag to enable/disable checking of tag names
If enabled, will raise
exiftool.exceptions.ExifToolTagNameError
if an invalid tag name is detected.Warning
ExifToolHelper only checks the validity of the Tag NAME based on a simple regex pattern.
It does not validate whether the tag name is actually valid on the file type(s) you’re accessing.
It does not validate whether the tag you passed in that “looks like” a tag is actually an option
It does support a “#” at the end of the tag name to disable print conversion
Please refer to ExifTool Tag Names documentation for a complete list of valid tags recognized by ExifTool.
Warning
While this property is provided to give callers an option to enable/disable tag names checking, it is generally NOT recommended to disable
check_tag_names
.If disabled, you could accidentally edit a file when you meant to read it.
Example:
get_tags("a.jpg", "tag=value")
will callexecute_json("-tag=value", "a.jpg")
which will inadvertently write to a.jpg instead of reading it!That said, if PH’s exiftool changes its tag name regex and tag names are being erroneously rejected because of this flag, disabling this could be used as a workaround (more importantly, if this is happening, please file an issue!).
- Getter:
Returns current setting
- Setter:
Enable or Disable the check
Note
This settings can be changed any time and will only affect subsequent calls
- Type:
bool
- Return type:
bool
- exiftool.ExifToolHelper.execute(*params, **kwargs)
Override the
exiftool.ExifTool.execute()
methodAdds logic to auto-start if not running, if
auto_start
== TrueAdds logic to str() any parameter which is not a str or bytes. (This allows passing in objects like Path _without_ casting before passing it in.)
- Raises:
ExifToolExecuteError – If
check_execute
== True, and exit status was non-zero- Parameters:
params (Any) –
- Return type:
Union[str, bytes]
- exiftool.ExifToolHelper.get_metadata(files, params=None)
Return all metadata for the given files.
- Parameters:
files (Union[str, List]) – Files parameter matches
get_tags()
params (list or None) – Optional parameters to send to exiftool
- Returns:
The return value will have the format described in the documentation of
get_tags()
.- Return type:
List
- exiftool.ExifToolHelper.get_tags(files, tags, params=None)
Return only specified tags for the given files.
- Parameters:
files (Any or List(Any) - see Note) –
File(s) to be worked on.
If a non-iterable is provided, it will get tags for a single item (str(non-iterable))
If an iterable is provided, the list is passed into
execute_json()
verbatim.
Note
Any files/params which are not bytes/str will be casted to a str in
execute()
.Warning
Currently, filenames are NOT checked for existence! That is left up to the caller.
Warning
Wildcard strings are valid and passed verbatim to exiftool.
However, exiftool’s wildcard matching/globbing may be different than Python’s matching/globbing, which may cause unexpected behavior if you’re using one and comparing the result to the other. Read ExifTool Common Mistakes - Over-use of Wildcards in File Names for some related info.
tags (str, list, or None) –
Tag(s) to read. If tags is None, or [], method will returns all tags
Note
The tag names may include group names, as usual in the format
<group>:<tag>
.params (Any, List[Any], or None) – Optional parameter(s) to send to exiftool
- Returns:
The format of the return value is the same as for
exiftool.ExifTool.execute_json()
.- Raises:
ValueError – Invalid Parameter
TypeError – Invalid Parameter
ExifToolExecuteError – If
check_execute
== True, and exit status was non-zero
- Return type:
List
- exiftool.ExifToolHelper.run()
override the
exiftool.ExifTool.run()
methodWill not attempt to run if already running (so no warning about ‘ExifTool already running’ will trigger)
- Return type:
None
- exiftool.ExifToolHelper.set_tags(files, tags, params=None)
Writes the values of the specified tags for the given file(s).
- Parameters:
files (Any or List(Any) - see Note) –
File(s) to be worked on.
If a non-iterable is provided, it will get tags for a single item (str(non-iterable))
If an iterable is provided, the list is passed into
execute_json()
verbatim.
Note
Any files/params which are not bytes/str will be casted to a str in
execute()
.Warning
Currently, filenames are NOT checked for existence! That is left up to the caller.
Warning
Wildcard strings are valid and passed verbatim to exiftool.
However, exiftool’s wildcard matching/globbing may be different than Python’s matching/globbing, which may cause unexpected behavior if you’re using one and comparing the result to the other. Read ExifTool Common Mistakes - Over-use of Wildcards in File Names for some related info.
tags (dict) –
Tag(s) to write.
Dictionary keys = tags, values = tag values (str or list)
If a value is a str, will set key=value
If a value is a list, will iterate over list and set each individual value to the same tag (
Note
The tag names may include group names, as usual in the format
<group>:<tag>
.Note
Value of the dict can be a list, in which case, the tag will be passed with each item in the list, in the order given
This allows setting things like
-Keywords=a -Keywords=b -Keywords=c
by passing intags={"Keywords": ['a', 'b', 'c']}
params (str, list, or None) – Optional parameter(s) to send to exiftool
- Returns:
The format of the return value is the same as for
execute()
.- Raises:
ValueError – Invalid Parameter
TypeError – Invalid Parameter
ExifToolExecuteError – If
check_execute
== True, and exit status was non-zero
- exiftool.ExifToolHelper.terminate(**opts)
Overrides the
exiftool.ExifTool.terminate()
method.Will not attempt to terminate if not running (so no warning about ‘ExifTool not running’ will trigger)
- Parameters:
opts – passed directly to the parent call
exiftool.ExifTool.terminate()
- Return type:
None